Logging In
The API call !login
logs in to a new Insights Platform
session.
Description
You can log in to the Dynamic API user interface with the URL:
https://[host]/[1010-version]/api/!login
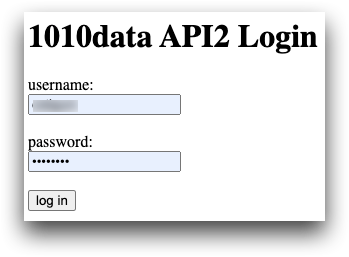
URL
https://[host]/[1010-version]/api/!login
Method
POST
Parameters
uid
- The User ID (required)
pw
- The password (required)
kill
- An optional parameter that determines whether you want to kill or
possess the existing session.
Possible values are as follows:
yes
- Terminate the existing session and start a new one.
possess
- Log in and possess the existing session.
Response
The following are possible login response codes:
- 200
- Login successful
A successful login returns the session cookie you need for future requests. The following is the JSON response from the
!login
endpoint:{'uid': 'userid', 'sid': sessionid, 'epw': 'encryptedpw'}
In addition, a
Set-Cookie
http header comes from the server.Set-Cookie
has the following format:Set-Cookie: session=userid|sessionid|encryptedpw
- 401
- Access denied
The user id and/or password are incorrect.
- 403
- Forbidden error
The user id and password combination has expired. The system displays a message suggesting you try logging in again.
- 405
- Method not allowed
You used a GET method instead of a POST method.
curl Example
The following example uses a curl command to test your login credentials. The
authentication cookie is then saved in the file cookie.txt
to be
used for subsequent commands.
$ curl -s -c cookie.txt -d uid=csmith -d pw=XXX
'https://www2.1010data.com/beta-latest/api/!login'
You can also use a JSON post to log in, as follows:
$ curl -s -c cookie.txt -H "Content-type: application/json" -X
POST -d '{"uid":"csmith", "pw":"XXX"}'
'https://www2.1010data.com/beta-latest/api/!login'
You will receive the following JSON response (note that the encrypted password below is truncated):
{"uid": "csmith", "sid": "956151005", "epw": "_______ca74fb26da1d29647b5cdc2acfb..."}
Python Example
The following Python 3 code logs into a Insights Platform session and returns a session cookie for future requests.
# Include system libraries import sys # standard python libs import json # for parsing api responses import getpass # for secure password entry import requests # Requests HTTP library for Python # http://python-requests.org/en/master/ # Prompt for username and password usr = input("Username: ") pwd = getpass.getpass() # The URL where we will post username and password credentials u0 = "https://www2.1010data.com/beta-latest/api/!login:json" # Send the POST request to the Dynamic API r = requests.post(u0, data=dict(uid=usr, pw=pwd), verify=True) # Decode the result so the sid and epw values may be stored and used in subsequent calls body = r.content.decode('utf-8') d = json.loads(body) session = dict(uid=usr, sid=int(d['sid']), epw=d['epw']) # Use the new session credentials to create a cookie, used in subsequent requests mycookie = {"session": "{uid}|{sid}|{epw}".format(**session)} # Display the session ID print("sessionID secured.\nsession cookie=:" + str(mycookie))
After you enter your user ID and password, this code returns the following JSON response (note that the encrypted password is truncated):
sessionID secured.
session cookie=:
{'session': 'csmith|956151005|_______491c5969cb2f8c6a8b43e2d300e3e6de4'}